Asp.net Encryption Key Generator
Hashing, Encryption and Random in ASP.NET Core. This post look at hashing, encryption and random string generation in ASP.NET Core. We examine a few different approaches and explain why some common techniques should be avoided in modern applications. Generating a random string. It is a very common requirement to generate random strings. May 13, 2009 The machineKey element of the ASP.NET web.config specifies the algorithm and keys that ASP.NET will use for encryption. By default the validationKey and the decryptionKey keys are set to AutoGenerate which means the runtime will generate a random key for use. This works fine for applications that are deployed on a single server. Generate ASP.NET Machine Keys This tool allows you to create a valid random machine key for validation and encryption/decryption of ASP.NET view state. This is beneficial in a webfarm where all of the server nodes need to have the same machine key, and it is also beneficial on a single box to keep the machine key consistent between IIS recycles and server reboots. Jul 21, 2017 I am using Asp.net membership in my application. We were using SHA256 for validation and 3DES for decryption (Asp.net membership) in machine key in Web.config. Now my requirement is to use AES encryption for both. I created Keys from IIS and added in my Web.config. What Is Machine Key? The machineKey element in the ASP.NET web.config file specifies the algorithm and keys that ASP.NET will use for encryption. By default the validationKey and the decryptionKey keys are set to AutoGenerate which means the runtime will generate a random key for use.
- This tool allows you to create a valid random machine key for validation and encryption/decryption of ASP.NET view state. This is beneficial in a webfarm where all of the server nodes need to have the same machine key, and it is also beneficial on a single box to keep the machine key consistent between IIS recycles and server reboots.
- Sep 05, 2006 Download Machine Key Generator. The Element configures keys to use for encryption and decryption of forms authentication cookie data and viewstate data, and for verification of out-of-process session state identification.
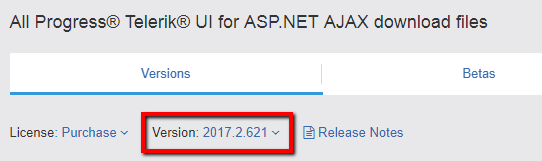
#regionEncryption |
/// <summary> |
/// Generate a private key |
/// From : www.chapleau.info/blog/2011/01/06/usingsimplestringkeywithaes256encryptioninc.html |
/// </summary> |
privatestaticstringGenerateKey(intiKeySize) |
{ |
RijndaelManagedaesEncryption=newRijndaelManaged(); |
aesEncryption.KeySize=iKeySize; |
aesEncryption.BlockSize=128; |
aesEncryption.Mode=CipherMode.CBC; |
aesEncryption.Padding=PaddingMode.PKCS7; |
aesEncryption.GenerateIV(); |
stringivStr=Convert.ToBase64String(aesEncryption.IV); |
aesEncryption.GenerateKey(); |
stringkeyStr=Convert.ToBase64String(aesEncryption.Key); |
stringcompleteKey=ivStr+','+keyStr; |
returnConvert.ToBase64String(ASCIIEncoding.UTF8.GetBytes(completeKey)); |
} |
/// <summary> |
/// Encrypt |
/// From : www.chapleau.info/blog/2011/01/06/usingsimplestringkeywithaes256encryptioninc.html |
/// </summary> |
privatestaticstringEncrypt(stringiPlainStr, stringiCompleteEncodedKey, intiKeySize) |
{ |
RijndaelManagedaesEncryption=newRijndaelManaged(); |
aesEncryption.KeySize=iKeySize; |
aesEncryption.BlockSize=128; |
aesEncryption.Mode=CipherMode.CBC; |
aesEncryption.Padding=PaddingMode.PKCS7; |
aesEncryption.IV=Convert.FromBase64String(ASCIIEncoding.UTF8.GetString(Convert.FromBase64String(iCompleteEncodedKey)).Split(',')[0]); |
aesEncryption.Key=Convert.FromBase64String(ASCIIEncoding.UTF8.GetString(Convert.FromBase64String(iCompleteEncodedKey)).Split(',')[1]); |
byte[] plainText=ASCIIEncoding.UTF8.GetBytes(iPlainStr); |
ICryptoTransformcrypto=aesEncryption.CreateEncryptor(); |
byte[] cipherText=crypto.TransformFinalBlock(plainText, 0, plainText.Length); |
returnConvert.ToBase64String(cipherText); |
} |
/// <summary> |
/// Decrypt |
/// From : www.chapleau.info/blog/2011/01/06/usingsimplestringkeywithaes256encryptioninc.html |
/// </summary> |
privatestaticstringDecrypt(stringiEncryptedText, stringiCompleteEncodedKey, intiKeySize) |
{ |
RijndaelManagedaesEncryption=newRijndaelManaged(); |
aesEncryption.KeySize=iKeySize; |
aesEncryption.BlockSize=128; |
aesEncryption.Mode=CipherMode.CBC; |
aesEncryption.Padding=PaddingMode.PKCS7; |
aesEncryption.IV=Convert.FromBase64String(ASCIIEncoding.UTF8.GetString(Convert.FromBase64String(iCompleteEncodedKey)).Split(',')[0]); |
aesEncryption.Key=Convert.FromBase64String(ASCIIEncoding.UTF8.GetString(Convert.FromBase64String(iCompleteEncodedKey)).Split(',')[1]); |
ICryptoTransformdecrypto=aesEncryption.CreateDecryptor(); |
byte[] encryptedBytes=Convert.FromBase64CharArray(iEncryptedText.ToCharArray(), 0, iEncryptedText.Length); |
returnASCIIEncoding.UTF8.GetString(decrypto.TransformFinalBlock(encryptedBytes, 0, encryptedBytes.Length)); |
} |
#endregion |
commented Jun 6, 2014
hi fairly new to the cryptography.. License key generator for pc games. For 2014 versions and later, you canfind them in Autodesk Account, on the Management tab. Serial Number LocationsSerial numbers are unique codes associated with your AutodeskAccount and a particular product that you have purchased or isotherwise available to you. please suggest a resolution |
commented Oct 9, 2017 • edited
edited
How-to save -safely- the private key ? Windows registry ? in disk ? I use ASP.NET applications. Test your code |
You may generate an RSA private key with the help of this tool. Additionally, it will display the public key of a generated or pasted private key.
Description
RSA is an asymmetric encryption algorithm. With a given key pair, data that is encrypted with one key can only be decrypted by the other. This is useful for encrypting data between a large number of parties; only one key pair per person need exist. RSA is widely used across the internet with HTTPS.
To generate a key pair, select the bit length of your key pair and click Generate key pair. Depending on length, your browser may take a long time to generate the key pair. A 1024-bit key will usually be ready instantly, while a 4096-bit key may take up to several minutes. For a faster and more secure method, see Do It Yourself below.
CryptoTools.net does not yet have a tool for facilitating the encryption and decryption of data using RSA, but you may Do It Yourself with the instructions below.
Do It Yourself
For these steps, you will need a command line shell with OpenSSL. Ideally, you should have a private key of your own and a public key from someone else. For demonstration, we will only use a single key pair.
Generate Private Key
Run this command to generate a 4096-bit private key and output it to the private.pem file. If you like, you may change the key length and/or output file.
Derive Public Key
Encryption Key Generator
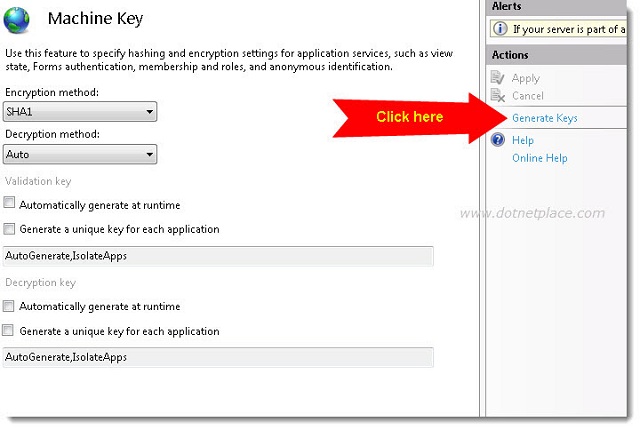
Given a private key, you may derive its public key and output it to public.pem using this command. (You may also paste your OpenSSL-generated private key into the form above to get its public key.)
Encrypt Data
We can now use this key pair to encrypt and decrypt a file, data.txt.
Decrypt Data
Encryption Key Example
Given the encrypted file from the previous step, you may decrypt it like so.