Generate Aes 256 Key Python
Symmetic encryption
Let me address your question about 'modes.' AES256 is a kind of block cipher.It takes as input a 32-byte key and a 16-byte string, called the block and outputs a block. We use AES in a mode of operation in order to encrypt. I wrote a simple algorithm to encrypt and decrypt files in Python using aes-256-cbc. From Crypto import Random from Crypto.Cipher import AES import base64 def pad(s): return s + b'0'. (AES. Technically, not as stated. AES-256 requires a 256bit key. SHA-512 will output 512 bits so unless you chop off half of the digest it will not work. A better solution is to use a standard and well tested key derivation function such as pbkdf2. Don't roll your own crypto unless absolutely necessary. Use vetted constructions. Apr 27, 2016 Encrypt data using AES and 256-bit keys AES stands for Advanced Encryption Standard and is an industry-standard algorithm for encrypting data symmetrically which even the US government has approved for SECRET documents.
For symmetic encryption, you can use the following:
To encrypt:
To decrypt:
Asymmetric encryption
For Asymmetric encryption you must first generate your private key and extract the public key.
To encrypt:
To decrypt:
Encripting files
You can't directly encrypt a large file using rsautl
. Instead, do the following:
- Generate a key using
openssl rand
, e.g.openssl rand 32 -out keyfile
. - Encrypt the key file using
openssl rsautl
. - Encrypt the data using
openssl enc
, using the generated key from step 1. - Package the encrypted key file with the encrypted data. The recipient will need to decrypt the key with their private key, then decrypt the data with the resulting key.
Ultimate solution for safe and high secured encode anyone file in OpenSSL and command-line:
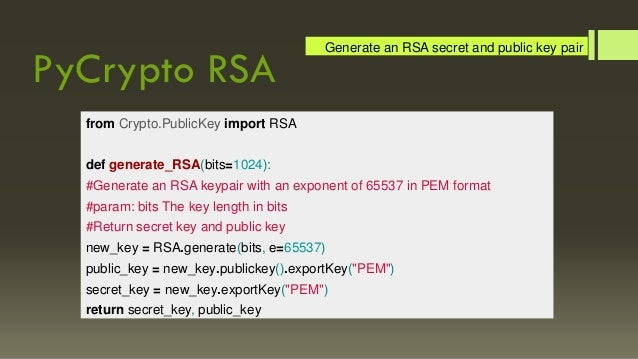
Private key generation (encrypted private key):
With unecrypted private key:
With encrypted private key:
With existing encrypted (unecrypted) private key:
Encrypt a file
Encrypt binary file:
Encrypt text file:
What is what:
smime
— ssl command for S/MIME utility (smime(1)).-encrypt
— chosen method for file process.-binary
— use safe file process. Normally the input message is converted to 'canonical' format as required by the S/MIME specification, this switch disable it. It is necessary for all binary files (like a images, sounds, ZIP archives).-aes-256-cbc
— chosen cipher AES in 256 bit for encryption (strong). If not specified 40 bit RC2 is used (very weak). (Supported ciphers).-in plainfile.zip
— input file name.-out encrypted.zip.enc
— output file name.-outform DER
— encode output file as binary. If is not specified, file is encoded by base64 and file size will be increased by 30%.yourSslCertificate.pem
— file name of your certificate's. That should be in PEM format.
That command can very effectively a strongly encrypt any file regardless of its size or format.
Decrypt a file
Decrypt binary file:
For text files:
What is what:
-inform DER
— same as-outform
above.-inkey private.key
— file name of your private key. That should be in PEM format and can be encrypted by password.-passin pass:your_password
— (optional) your password for private key encrypt.
Verification

Creating a signed digest of a file:
During these challenging times, we guarantee we will work tirelessly to support you. Activate windows xp by phone key generator. We will get through this together.Sincerely,Elizabeth DouglasCEO, wikiHow. Thank you to our community and to all of our readers who are working to aid others in this time of crisis, and to all of those who are making personal sacrifices for the good of their communities. We will continue to give you accurate and timely information throughout the crisis, and we will deliver on our mission — to help everyone in the world learn how to do anything — no matter what. But we are also encouraged by the stories of our readers finding help through our site.
Verify a signed digest:
Source
Latest versionReleased:
A Python implementation of OpenPGP
Project description
Summary
python-pgp aims to reproduce the full functionality of GnuPG in Python.It may also be used for creating raw OpenPGP packets and packet streamsfor test purposes. This may be a bit of a heavyweight solution for somepurposes.
This is a fork of the original library - the original one does not seem to be active and/or have a PyPI package.
Alternatives
Other Python packages which provide related functionality:
- pyassuan - communicatewith GnuPG using its socket protocol.
- pgpdump - a pure pythonlibrary for parsing OpenPGP packets.
- gnupg - a wrapper around theGnuPG executable.
- python-gnupg - anotherwrapper around the GnuPG executable.
- gpgkeys - another wrapperaround the GnuPG executable.
- gpglib - a pure pythonlibrary for parsing OpenPGP packets and decrypting messages.
- OpenPGP - an unmaintainedpure python library with much of the functionality of old versionsof GnuPG.
- encryptedfile - apure python library for symmetrically encrypting files in anOpenPGP-compatible way.
- PGPy - a pure pythonlibrary with basic parsing and signing of OpenPGP packets.
- OpenPGP-Python - apure python port ofopenpgp-php. It canparse OpenPGP packets and verify & create signatures.
System requirements
- build-essential
Recommended
- libgmp10-dev (for fastmath extension of pycrypto)
Installation
with Twofish support:
with Camellia support:
with Twofish & Camellia support:
Usage
Generate Aes 256 Key Python Key
High level
Parsing a transferrable key
Retrieving a key from a keyserver and creating a message for it
Low level
Serializing a packet
Security
If you are using this package to handle private key data anddecryption, please note that there is no (reasonable) way currently inPython to securely erase memory and that copies of things are made oftenand in non-obvious ways. If you are concerned about key data beingcompromised by a memory leak, do not use this package for handlingsecret key data. On the other hand, “if your memory is constantly beingcompromised, I would re-think your security setup.”
OpenPGP uses compression algorithms. Beware when feeding untrusted datainto this library ofZip bomb or similar denialof service attacks.
Development
The main repository for this package is on GitHub. To develop on the packageand install development dependencies, clone the repository and installthe ‘dev’ extras.:
Building documentation
License
Copyright (C) 2014 Richard Mitchell
Generate Aes Key Python
This program is free software: you can redistribute it and/or modifyit under the terms of the GNU General Public License as published bythe Free Software Foundation, either version 3 of the License, or(at your option) any later version.
This program is distributed in the hope that it will be useful,but WITHOUT ANY WARRANTY; without even the implied warranty ofMERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See theGNU General Public License for more details.
You should have received a copy of the GNU General Public Licensealong with this program. If not, see <http://www.gnu.org/licenses/>.
Release historyRelease notifications
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size py_pgp-0.0.1-py3.2.egg (295.3 kB) | File type Egg | Python version 3.2 | Upload date | Hashes |
Filename, size py_pgp-0.0.1-py3.3.egg (381.9 kB) | File type Egg | Python version 3.3 | Upload date | Hashes |
Filename, size py_pgp-0.0.1-py3.4.egg (375.8 kB) | File type Egg | Python version 3.4 | Upload date | Hashes |
Filename, size py_pgp-0.0.1-py3-none-any.whl (172.9 kB) | File type Wheel | Python version 3.4 | Upload date | Hashes |
Filename, size py-pgp-0.0.1.tar.gz (126.0 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for py_pgp-0.0.1-py3.2.egg
Algorithm | Hash digest |
---|---|
SHA256 | 3bf921781fdef4b8a9bc83cf2a76782cbd3446b4c96e4f4d2edb81821b8bad4d |
MD5 | 38037cacb2660a9ff7683043c84b8cd4 |
BLAKE2-256 | 3abbc77504dfa1847310182f3c165ef08256c1819a1325040ef2fffd6ad1ba70 |
Hashes for py_pgp-0.0.1-py3.3.egg
Algorithm | Hash digest |
---|---|
SHA256 | ed96b27acf35ff69c8902364594429eb0d83e95bec5157b544fc21630af4d5eb |
MD5 | b4b77fb556072eb94d652b719b2c50b4 |
BLAKE2-256 | b28847f5a918c196f2b956c22d493f6f1ca1827d8f3ecd81d602139045f99aa7 |
Hashes for py_pgp-0.0.1-py3.4.egg
Algorithm | Hash digest |
---|---|
SHA256 | 1c683e4cbae84f4f5500714493bbdb2845238bd4860e61cb5485b7a1094fd14f |
MD5 | b02ced06a077a8d539a73503021d4f44 |
BLAKE2-256 | 66ad8da6e200632a3232ae59f0ef7bd24a611263fb20cc6028f31923158b10ca |
Hashes for py_pgp-0.0.1-py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | e3d2b7bdf73d8e97cca12abad484df4d14d6b08da206f9547b839ff702a4ff83 |
MD5 | dd7242bccdb855db099e077940d67f47 |
BLAKE2-256 | 473a13a9d55dabdb316d82017014553e97ebc11e833c07615a5b24930acf01ae |
Hashes for py-pgp-0.0.1.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | cad3611491598d1111a8abe0ebe5aa55119353bd8f7c78f623c16d131d3b85fc |
MD5 | 3a20198672730d10fd35bd18047023a6 |
BLAKE2-256 | 63d142ae81ae33db41b7474df650c71ea7648a14477a8103d53c974c872a24b8 |