Openssl Aes Key Generation C++
Cryptogams is Andy Polyakov's project used to develop high speed cryptographic primitives and share them with other developers. This wiki article will show you how to use Cryptogams ARMv4 AES implementation. According to the head notes the ARMv4 implementation runs. Apr 17, 2015. Create a 256 bit key and IV using the supplied keydata. Salt can be added for taste. Fills in the encryption and decryption ctx objects and returns 0 on success int aesinit ( unsigned char.keydata, int keydatalen, unsigned char.salt, EVPCIPHERCTX.ectx. Here i use AES-128 bit CBC mode Encryption, where 128 bit is AES key length. We can also use 192 and 256 bit AES key for encryption in which size and length of key is increased with minor modification in following code. AES Sample code in C programming.
- Aes Key Fortnite
- Openssl Aes Key Generation C 1
- Openssl Aes Key Generation
- Openssl Aes Key Generation C Definition
The EVP functions support the ability to generate parameters and keys if required for EVPPKEY objects. Since these functions use random numbers you should ensure that the random number generator is appropriately seeded as discussed here.
Cryptogams is Andy Polyakov's project used to develop high speed cryptographic primitives and share them with other developers. This wiki article will show you how to use Cryptogams ARMv4 AES implementation. According to the head notes the ARMv4 implementation runs around 22 to 40 cycles per byte (cpb). Typical C/C++ implementations run around 50 to 80 cpb and Andy's routines should outperform all of them.
Andy's Cryptogam implementations are provided by OpenSSL, but they are also available stand alone under a BSD license. The BSD style license is permissive and allows developers to use Andy's high speed cryptography without an OpenSSL dependency or licensing terms.
There are 6 steps to the process. The first step obtains the sources. The second step creates an ASM source file. The third step compiles and assembles the source file into an object file. The fourth steps determines the API. The fifth step creates a C header file. The final step integrates the object file into a program. Once you create the files aes-armv4.h and aes-armv4.S you can use sed to restore symbols back to their Cryptogams name with sed -i 's OPENSSL CRYPTOGAMS g' aes-armv4.h aes-armv4.S.
A few cautions before you begin. First, you are going to examine undocumented features of the OpenSSL library to learn how to work with the Cryptogam's sources. The Cryptogam sources are stable but things could change over time. Second, the ARMv4 implementation operates in ECB mode and encrypts or decrypts full AES blocks. You are responsible for things like padding and side channel counter-measures.
At the moment Clang is miscompiling aes-armv4.S. Also see LLVM Issue 38133. You can work around the problem by compiling aes-armv4.S with -mthumb, but all data must be aligned. If you don't use aligned buffers then a SIGBUS could occur.
Obtain Source Files[edit]
There are two source files you need for Cryptogams AES. The first is arm-xlate.pl and the second is aes-armv4.pl. They are available in the OpenSSL sources. The following commands fetch OpenSSL and then peels off the two Cryptogams files of interest.
Create ASM File[edit]
The second step is to run aes-armv4.pl to produce an assembly language source file that can be consumed by GCC. aes-armv4.pl internally calls arm-xlate.pl. linux32 is the flavor used by the translate program. aes-armv4.S is the output filename. In the command below note the *.S file extension, which is a capitol S. Do not use a lowercase s because GCC must drive the compile and assemble step.
GCC is needed to drive the process because there are C macros in the source file. Some Cryptogam source files have this requirement, while some others do not. aes-armv4 happens to have the requirement.
At this point there is an ASM file but it needs two small fixups. First, arm_arch.h is an OpenSSL source file so the dependency must be removed. Second, GCC defines __ARM_ARCH instead of __ARM_ARCH__ so a sed is needed.
To fixup the source files execute the following two commands:
Alternately, instead of the two sed's, you can open arm_arch.h, copy the defines and paste them directly into aes-armv4.S. Take care when using arm_arch.h as it carries the OpenSSL license.
After the two fixups aes-armv4.S is ready to be compiled by GCC.
Compile Source File[edit]
The source file is ready to be compiled and assembled. At this point there are two choices. First, you can use ARMv5t or higher which includes Thumb instructions. The following compiles the source file with ARMv5t.
The second choice uses ARMv4 and avoids Thumb instructions. If you want to avoid Thumb then add -marm to you compile command.
Using ARMv5t as an example you now have an object file with the following symbols. Symbols with a capitol T are public and exported. Symbols with a lower t are private and should not be used.
And you can inspect the generated code with objdump.
And trace it back to the source code in aes-armv4.S.
Determine API[edit]
The next step is determine the API so you can call it from a C program. Unfortunately the API is not documented and you have to dig around the OpenSSL sources. The functions of interest are AES_set_encrypt_key, AES_set_decrypt_key, AES_encrypt and AES_decrypt.
A quick grep of OpenSSL sources reveals the following for AES_set_encrypt_key.
Examining aes_core.c:632 reveals the following.
The next piece of information to discover is AES_KEY. Again a quick grep leads you to aes_key_st.
Finally, you need AES_MAXNR from aes.h.
Lather, rinse repeat for AES_set_decrypt_key, AES_encrypt and AES_decrypt. AES_encrypt can be found at crypto/aes/aes_core.c:787.
And AES_decrypt can be found at aes_core.c:978.
Create C Header[edit]
The fifth step creates a C header file based on information from Determine API. The header file is needed for two reasons. First, it removes the OpenSSL dependency from your project. Second, it avoids OpenSSL licensing violations.
Below is the C Header file you can use.
Test Program[edit]
The final step is to test the integration of Cryptogam's AES with your program.
Aes Key Fortnite
And the test program is shown below.
Symbol Names[edit]
The article used the same names as they appeared in the Cryptogams source code. For example, AES_set_encrypt_key and AES_set_decrypt_key are the names of two functions in the source code, and they will show up in the object file and when compiled and the library when linked.
It is possible the function and date names will collide if you also link to OpenSSL, either directly or indirectly. If you plan on using Cryptogams code in a shared object then you should rename all symbols to avoid collisions. To rename symbols for AES you should rename all AES_* names. Assuming you are using MYLIB as a prefix the following sed should do the job.
You can verify public symbols were renamed with nm aes-armv4.o. Generally speaking, all symbols with capitol letters like T (public function), B (uninitialized data), C (common data), D (initialized data), and R (read-only data) should be renamed.
Benchmarks[edit]
You can perform a rough benchmark using the code shown below. Prior to executing the benchmark program you should move the CPU from on-demand or powersave to performance mode.
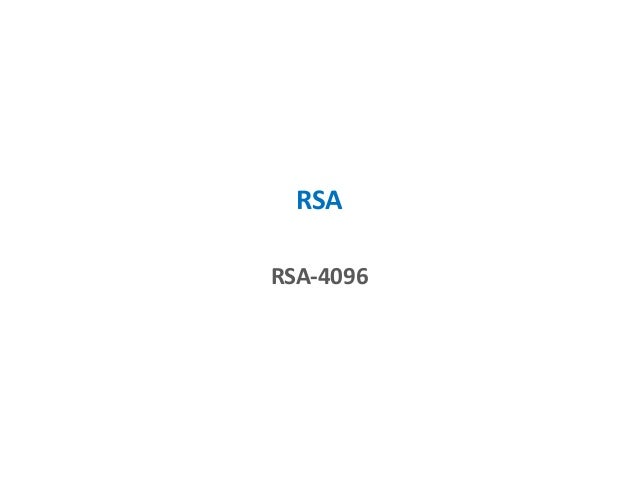
The results below are from a BananaPi with a Cortex-A7 Sun7i SoC running at 950 MHz. A C/C++ AES implementation runs about 65 cpb on the dev-board. Notice aes-armv4.S was compiled with -march=armv7.
And the following is from a Wandboard Dual with a NXP i.MX6 Cortex-A9 running at 1 GHz. A C/C++ implementation runs around 40 cpb.
Autotools[edit]
If you are using Autotools you can add the following to configure.ac and Makefile.am to conditionally compile aes-armv4.S for A-32 platforms. You will need to detect ARM A-32 and set IS_ARM32 to non-0. Also see Automake Assembly Support in section 8.13 of the manual.
Openssl Aes Key Generation C 1
First, the configure.ac recipe:
Openssl Aes Key Generation
Second, the Makefile.am recipe: